在Java中可以使用Java 8的java.time API的 `Period.between()` 函数来计算两个日期之间的差距,包括相差的月份。
一、使用Period.between()方法
Java 8中的java.time API提供了`Period`类,这个类有一个 `between` 方法可以计算两个日期之间的差距。`Period`类提供了 `getMonths()` 和 `getYears()` 方法,可以获取两个日期间相差的月份和年份。
import java.time.LocalDate;
import java.time.Period;
public class Main {
public static void main(String[] args) {
LocalDate date1 = LocalDate.of(2018, 7, 12);
LocalDate date2 = LocalDate.of(2021, 9, 21);
Period period = Period.between(date1, date2);
int diff = period.getYears() * 12 + period.getMonths();
System.out.println("Between " + date1 + " and " + date2 + ", there is a difference of " + diff + " months.");
}
}
二、不使用内置方法
除了使用Java API提供的方法计算月份差之外,还可以通过获取两个日期的年和月,然后计算出两个日期间的月份。这种方法虽然较为繁琐,但是在旧版本的Java中,可能没有API提供的方法方便和直观。
import java.util.Calendar;
import java.util.Date;
import java.util.GregorianCalendar;
public class Main {
public static void main(String[] args) {
Calendar cal1 = new GregorianCalendar(2021, 9, 21);
Calendar cal2 = new GregorianCalendar(2018, 7, 12);
int monthsDiff = (cal1.get(Calendar.YEAR) - cal2.get(Calendar.YEAR)) * 12 +
(cal1.get(Calendar.MONTH) - cal2.get(Calendar.MONTH));
System.out.println("Between " + cal1.getTime() + " and " + cal2.getTime() + ", there is a difference of "
+ monthsDiff + " months.");
}
}
织梦狗教程
本文标题为:Java计算两个日期相差几个月
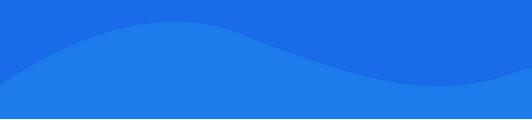
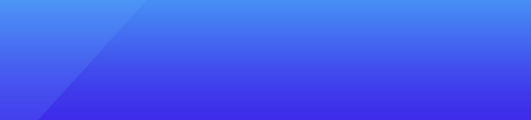
基础教程推荐
猜你喜欢
- Project Reactor源码解析publishOn使用示例 2023-04-12
- SpringBoot配置文件中密码属性加密的实现 2023-03-11
- Java去掉小数点后面无效0的方案与建议 2023-02-18
- Java File类的概述及常用方法使用详解 2023-05-18
- 一文了解Java 线程池的正确使用姿势 2023-06-17
- Java使用EasyExcel进行单元格合并的问题详解 2023-01-18
- 用java实现扫雷游戏 2022-12-06
- JVM分析之类加载机制详解 2023-04-06
- 全局记录Feign的请求和响应日志方式 2023-01-09
- 工厂方法在Spring框架中的运用 2023-06-23