JavaMail是一个通过邮件服务器发送和接收邮件的平台独立的框架。
一、简单邮件发送
首先我们需要创建一个Session对象,然后创建一个默认的MimeMessage对象。
import javax.mail.*;
import javax.mail.internet.*;
import java.util.Properties;
public class EmailSender {
public void sendEmail(String recipient, String subject, String messageBody) {
//定义发送邮件的属性
final String username = "your-email-id";
final String password = "your-password";
Properties props = new Properties();
props.put("mail.smtp.auth", "true");
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.host", "smtp.gmail.com");
props.put("mail.smtp.port", "587");
//获取Session对象
Session session = Session.getInstance(props,
new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(username, password);
}
});
try {
//创建MimeMessage对象
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress("from-email"));
message.setRecipients(Message.RecipientType.TO,
InternetAddress.parse(recipient));
message.setSubject(subject);
message.setText(messageBody);
//发送邮件
Transport.send(message);
} catch (MessagingException e) {
throw new RuntimeException(e);
}
}
}
二、发送含有附件的邮件
如果你想要在邮件中添加附件,则需要创建一个使用Multipart实例的MimeMessage,并添加至少一个BodyPart实例到这个Multipart实例中。
public class EmailSenderWithAttachment {
public void sendEmailWithAttachment(String recipient, String subject, String messageBody, String fileName) {
//创建和配置Session
//...省略相同的部分代码...
try {
//创建含有附件的邮件
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress("from-email"));
message.setRecipients(Message.RecipientType.TO,InternetAddress.parse(recipient));
message.setSubject(subject);
Multipart multipart = new MimeMultipart();
BodyPart messageBodyPart = new MimeBodyPart();
messageBodyPart.setText(messageBody);
multipart.addBodyPart(messageBodyPart);
//创建附件部分
messageBodyPart = new MimeBodyPart();
DataSource source = new FileDataSource(fileName);
messageBodyPart.setDataHandler(new DataHandler(source));
messageBodyPart.setFileName(fileName);
multipart.addBodyPart(messageBodyPart);
//设置邮件内容
message.setContent(multipart);
//发送邮件
Transport.send(message);
} catch (MessagingException e) {
throw new RuntimeException(e);
}
}
}
这段代码中添加了一个新的MimeBodyPart对象到Multipart实例中,这个新的对象包含了附件的内容
织梦狗教程
本文标题为:使用Java发送邮件
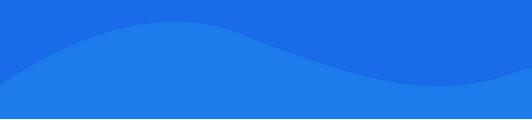
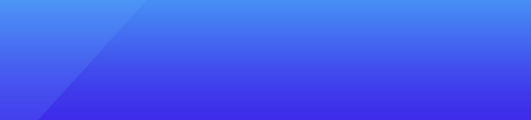
基础教程推荐
猜你喜欢
- 一文了解Java 线程池的正确使用姿势 2023-06-17
- 全局记录Feign的请求和响应日志方式 2023-01-09
- SpringBoot配置文件中密码属性加密的实现 2023-03-11
- 用java实现扫雷游戏 2022-12-06
- Java File类的概述及常用方法使用详解 2023-05-18
- 工厂方法在Spring框架中的运用 2023-06-23
- JVM分析之类加载机制详解 2023-04-06
- Java去掉小数点后面无效0的方案与建议 2023-02-18
- Project Reactor源码解析publishOn使用示例 2023-04-12
- Java使用EasyExcel进行单元格合并的问题详解 2023-01-18