Java CompletableFuture是Java中的异步编程工具,是Future的加强版,其可以用于异步执行任务,它提供了更强大的扩展性和灵活性,可以灵活组装和处理多个Future。
一、Java CompletableFuture的基本使用
Java CompletableFuture可以很容易地创建异步任务,你只需要提供一个Supplier函数型接口的lambda表达式即可。
下面是创建和获取CompletableFuture的结果的示例代码:
CompletableFuture future = CompletableFuture.supplyAsync(() -> {
// 模拟复杂计算任务
try {
Thread.sleep(2000);
} catch (InterruptedException e) {
throw new IllegalStateException(e);
}
// 返回计算结果
return "Result";
});
而对于结果的获取,我们有多种方式,下面将展示阻塞和非阻塞两种方式:
// 阻塞方式:get
try {
String result = future.get(); //此处会阻塞,等待结果的产生
System.out.println(result);
} catch (Exception e) {
throw new IllegalStateException(e);
}
// 非阻塞方式:thenApply
future.thenApply(result -> {
System.out.println(result);
return null;
});
二、Java CompletableFuture的组合使用
除了基本的使用,Java CompletableFuture最大的优点在于其“组合性”,这一点主要体现在它有一系列的方法可以对多个CompletableFuture进行组合。
下面是一个CompletableFuture的组合使用的示例:
CompletableFuture future1 = CompletableFuture.supplyAsync(() -> "Hello");
CompletableFuture future2 = CompletableFuture.supplyAsync(() -> "World");
CompletableFuture future = future1.thenCombine(future2, (s1, s2) -> s1 + " " + s2);
// 输出: Hello World
System.out.println(future.get());
三、Java CompletableFuture的异常处理
Java CompletableFuture提供了一些异常处理的方法,可以很方便地处理在异步任务执行中产生的异常。
下面是使用异常处理的示例:
CompletableFuture future = CompletableFuture.supplyAsync(() -> {
if (true) {
throw new RuntimeException("Exception happened.");
}
return "Result";
});
// 使用exceptionally处理异常
future = future.exceptionally(e -> "Default Value");
// 输出: Default Value
System.out.println(future.get());
上述例子中,我们故意抛出一个异常,然后使用exceptionally方法来处理这个异常,如果任务执行成功,这个函数不会被执行,如果抛出异常,就会走这个异常处理逻辑,这个函数的返回值会被正常处理。
本文标题为:理解Java CompletableFuture
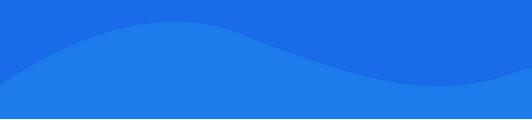
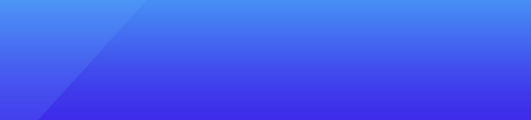
基础教程推荐
- 用java实现扫雷游戏 2022-12-06
- SpringBoot配置文件中密码属性加密的实现 2023-03-11
- JVM分析之类加载机制详解 2023-04-06
- 一文了解Java 线程池的正确使用姿势 2023-06-17
- 全局记录Feign的请求和响应日志方式 2023-01-09
- 工厂方法在Spring框架中的运用 2023-06-23
- Project Reactor源码解析publishOn使用示例 2023-04-12
- Java使用EasyExcel进行单元格合并的问题详解 2023-01-18
- Java去掉小数点后面无效0的方案与建议 2023-02-18
- Java File类的概述及常用方法使用详解 2023-05-18