SpringBoot对redis非常友好,不需要像传统项目那样使用Jedis,只要添加spring-boot-starter-data-redis的maven依赖dependencygroupIdorg.springframework.boot/groupIdartifactIdspring-boot-starter-data-r...

SpringBoot对redis非常友好,不需要像传统项目那样使用Jedis,只要添加spring-boot-starter-data-redis的maven依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
接下来是添加redis和RedisTemplate的bean
package com.example.demo.config;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.PropertyAccessor;
import com.fasterxml.jackson.databind.ObjectMapper;
import org.springframework.cache.annotation.CachingConfigurerSupport;
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.cache.interceptor.KeyGenerator;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.serializer.Jackson2JsonRedisSerializer;
import java.lang.reflect.Method;
/**
* @Description:
* @Author: zhaowang
* @Date: 2018/10/8 15:42
* @Version: 1.0
*/
@Configuration
@EnableCaching
public class RedisConfig extends CachingConfigurerSupport {
@Bean
public KeyGenerator keyGenerator() {
return new KeyGenerator() {
@Override
public Object generate(Object target, Method method, Object... params) {
StringBuilder sb = new StringBuilder();
sb.append(target.getClass().getName());
sb.append(method.getName());
for (Object obj : params) {
sb.append(obj.toString());
}
return sb.toString();
}
};
}
@Bean(name = "redisTemplate")
public RedisTemplate<?, ?> redisTemplate(RedisConnectionFactory factory) {
StringRedisTemplate template = new StringRedisTemplate(factory);
Jackson2JsonRedisSerializer jackson2JsonRedisSerializer = new Jackson2JsonRedisSerializer(Object.class);
ObjectMapper om = new ObjectMapper();
om.setVisibility(PropertyAccessor.ALL, JsonAutoDetect.Visibility.ANY);
om.enableDefaultTyping(ObjectMapper.DefaultTyping.NON_FINAL);
jackson2JsonRedisSerializer.setObjectMapper(om);
template.setValueSerializer(jackson2JsonRedisSerializer);
template.afterPropertiesSet();
return template;
}
}
application.yml配置
spring:
redis:
#redis数据库索引(默认为0)
database: 0
#redis服务器地址
host: ip
#redis服务器连接端口
port: 6379
#redis连接密码
password: 123456
接下来是使用SpringBoot注解实现redis的存取操作的实例
因为使用了Spring Data Jpa,所以这里给出repository层的代码,然后在service层调用该层方法。
package com.example.demo.dao;
import com.example.demo.entity.DatabusEntity;
import org.springframework.cache.annotation.CacheConfig;
import org.springframework.cache.annotation.CacheEvict;
import org.springframework.cache.annotation.CachePut;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.data.jpa.repository.Modifying;
import org.springframework.data.jpa.repository.Query;
import org.springframework.data.repository.query.Param;
import org.springframework.stereotype.Repository;
import org.springframework.transaction.annotation.Transactional;
import java.util.List;
import java.util.Map;
/**
* @Description:
* @Author: zhaowang
* @Date: 2018/9/21 17:22
* @Version: 1.0
*/
@Repository
@CacheConfig(cacheNames = "databusEntity")
public interface DatabusDao extends JpaRepository<DatabusEntity, Integer> {
@Cacheable(key = "#p0", keyGenerator = "myKeyGenerator")
public DatabusEntity findById(int id);
@CachePut(key = "#p0.id") // 缓存插入的结果
@Override
@Transactional(value="transactionManager")
public DatabusEntity save(DatabusEntity databusEntity);
@CacheEvict(key="#p0", allEntries=true) // 删除对应的缓存
@Transactional(value="transactionManager")
public void deleteById(int id);
}
注解说明
@CacheConfig(cacheNames = "databusEntity")
cacheNames 指定该类所有方法操作的缓存名称,是全局的。也可以在方法上加,但缓存名称仅对该方法生效,是局部的。
@Cacheable(key = "#p0", keyGenerator = "myKeyGenerator")
1.@Cacheable : 如果方法的第一个参数key(p0)存在于redis缓存中,则从缓存中获取value作为方法的返回结果,否则按照方法定义获取返回结果(一般是查询数据库)。
2.#p0表示第一个参数的key,即保存该方法操作的缓存的key(以HashMap的形式存储)到第一个参数,keyGenerator 定义Key生成的类。
@CachePut(key = "#p0.id")
p0.id表示将第一个参数的id作为key缓存到redis中,value为第一个参数值。
@CacheEvict(key="#p0", allEntries=true)
1.p0表示删除key为第一个参数的redis缓存数据
2.allEntries=true表示删除缓存中的所有值(key和value)
allEntries=false表示仅删除key对应的value
测试类
package com.example.demo.controller;
import com.example.demo.dao.DatabusDao;
import com.example.demo.domain.DatabusTaskData;
import com.example.demo.entity.DatabusEntity;
import com.example.demo.service.DatabusService;
import com.example.demo.service.DatabusTaskDataService;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.*;
/**
* @Description: test
* @Author: zhaowang
* @Date: 2018/8/7 17:46
* @version: 1.0
*/
@Slf4j
@RestController
@RequestMapping("demo/con")
public class TestController {
@Autowired
private DatabusTaskDataService databusTaskDataService;
@Autowired
private DatabusService databusService;
@Autowired
private DatabusDao databusDao;
@RequestMapping("/cache")
public void cache() {
DatabusEntity databusEntity = databusDao.findById(3073);
System.out.println("第一次执行:"+databusEntity.getFile_name());
databusEntity.setFile_name("file1");
databusDao.save(databusEntity);
DatabusEntity databusEntity2 = databusDao.findById(3073);
System.out.println("第二次执行:"+databusEntity2.getFile_name());
databusDao.deleteById(3073);
DatabusEntity databusEntity3 = databusDao.findById(3073);
if(databusEntity3 != null)
System.out.println("第三次执行:"+databusEntity3.getFile_name());
}
}
访问url,对比结果可以清晰地知道redis的缓存put和evict的过程。
本文标题为:SpringBoot集成Redis和RedisTemplate
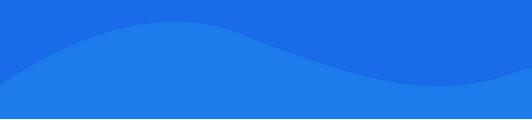
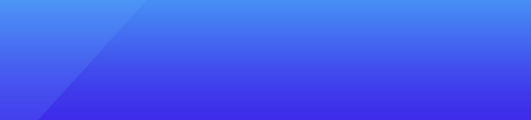
基础教程推荐
- Java程序员从笨鸟到菜鸟(五十三) 分布式之 Redis 2023-09-11
- Python安装第三方库的方法(pip/conda、easy_install、setup.py) 2023-07-28
- redis 数据库 2023-09-13
- Windows10系统中Oracle完全卸载正确步骤 2023-07-24
- Python常见库matplotlib学习笔记之画图中各个模块的含义及修改方法 2023-07-27
- Mariadb数据库主从复制同步配置过程实例 2023-07-25
- SQL Server如何设置用户只能访问特定数据库和访问特定表或视图 2023-07-29
- oracle19c卸载教程的超详细教程 2023-07-23
- redis乐观锁与悲观锁的实战 2023-07-13
- oracle数据库排序后如何获取第一条数据 2023-07-24